In this article, we’re going to program a PLC using both Ladder Diagram (LD) and C++. The LD program will accomplish simple Motor Start and Stop operations. The C++ program will produce logging data of motor activations.
We’ll use Phoenix Technology PLCnext Engineer to create the LD program, and Eclipse IDE to create the C++ project. PLCnext Engineer is used to run both the LD and C++ programs.
We will run our project utilizing the hardware and I/O on the PLCnext Starterkit.
Background information
Let’s start with a bit of background information.
The five programming languages specified in the IEC 61131-3 Standard are Ladder Diagram, Instruction List, Function Block Diagram, Structured Text, and Sequential Function Chart.
Most PLC Programming software is capable of programming using 2 or more of IEC 61131-3 Standard languages.
Generally speaking, PLC Programming software is not capable of higher-level language programming such as C++. C++ programs are created using other programming software.
The resulting C++ program can be added to or work alongside the PLC programming software allowing the IEC 61131-3 program and the C++ program to run together.
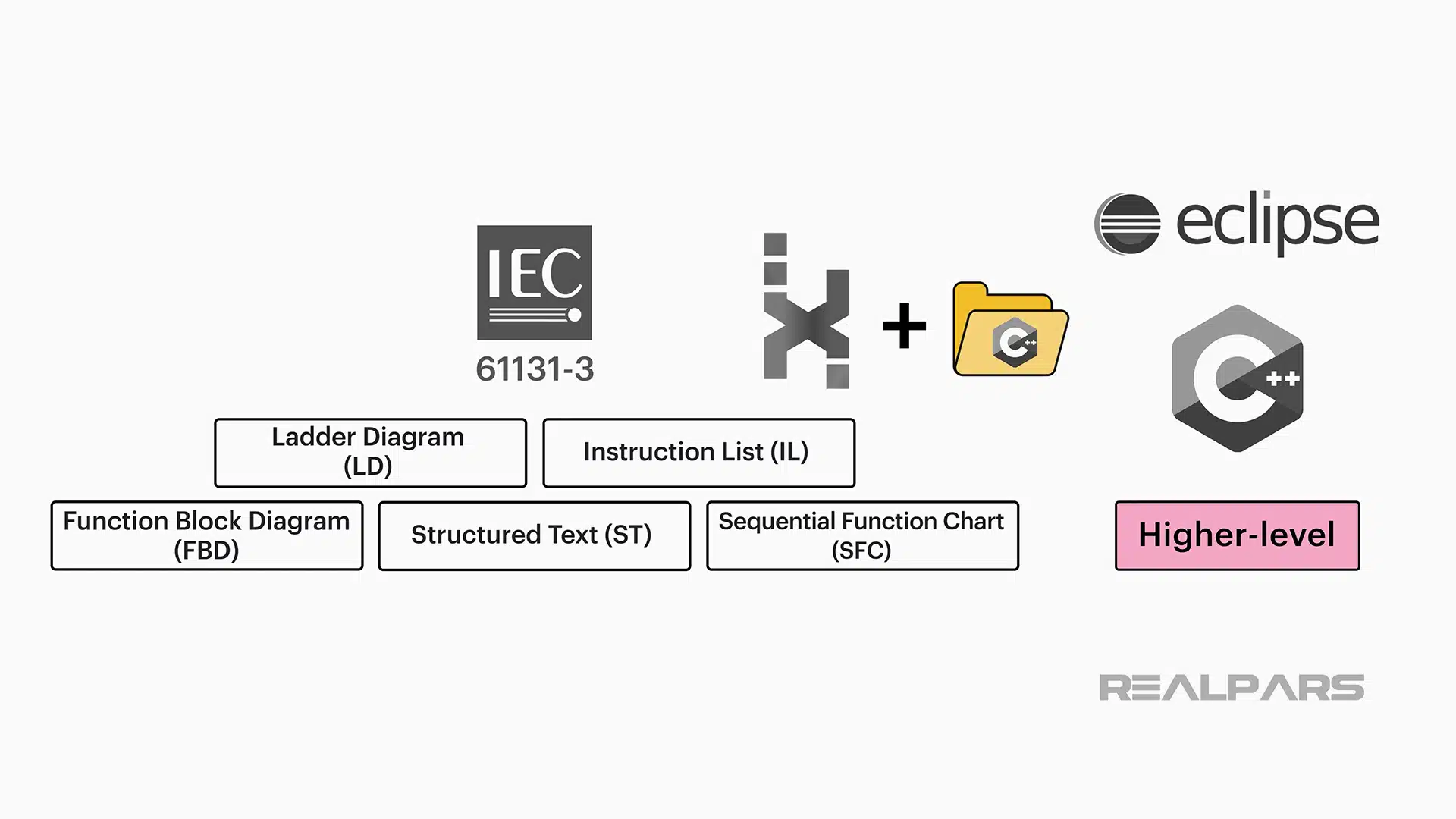
You might ask… Why do we use or need higher-level language programs in our PLC projects?
Well, the quick answer is that complex functions are not easily accomplished with traditional IEC 61131-3 languages.
For example, C++ programs can be easily created to produce log data to assist with Predictive Maintenance (PdM). A program of that complexity cannot be easily created using IEC 61131-3 languages such as Ladder Diagram.
So, how do we create C++ programs?
We can use a tool called an Integrated Development Environment or IDE for short. An IDE is a complete package that among other things, offers a code editor.
There are lots of C++ IDEs. Microsoft Visual Studio and Eclipse are 2 very commonly used IDE Editors. Eclipse is one of the simplest and that’s the Editor we use.
Ladder Diagram program
Let’s begin with a coded LD program written to operate a Motor.
The LD program has a START variable and a STOP variable assigned to our IN01 and IN02 inputs of the PLC digital input module.
Physical push-button normally-open switches are hard-wired to the IN01 and IN02 terminals of the PLC digital input module.

We’ve got a MOTOR variable assigned to our OUT01 output of the PLC digital output module.

The ladder diagram tells us that the MOTOR will run when we press the START switch and continue to run until we press the STOP switch.

C++ program
Ok… Let’s have a look at the C++ program that will produce logging data of motor activations.
As we said earlier, the C++ project is written using Eclipse IDE Editor.
Inside the Eclipse IDE, every C++ project folder is available via Project Explorer.
Opening the MotorStartStop folder will reveal several files and subfolders.
We’re going to discuss 2 of the files in the src folder; MotorStartStopProgram.cpp and MotorStartStopProgram.hpp.
The actual C++ program is written in these 2 text-based files.

C++ header file
We’ll start with the MotorStartStopProgram.hpp file.
We’ve created an Input port called Motor and an Output port called OUTPORT_MotorStarts.
Motor is a Boolean type and OUTPORT_MotorStarts is an int16 or DINT data type.
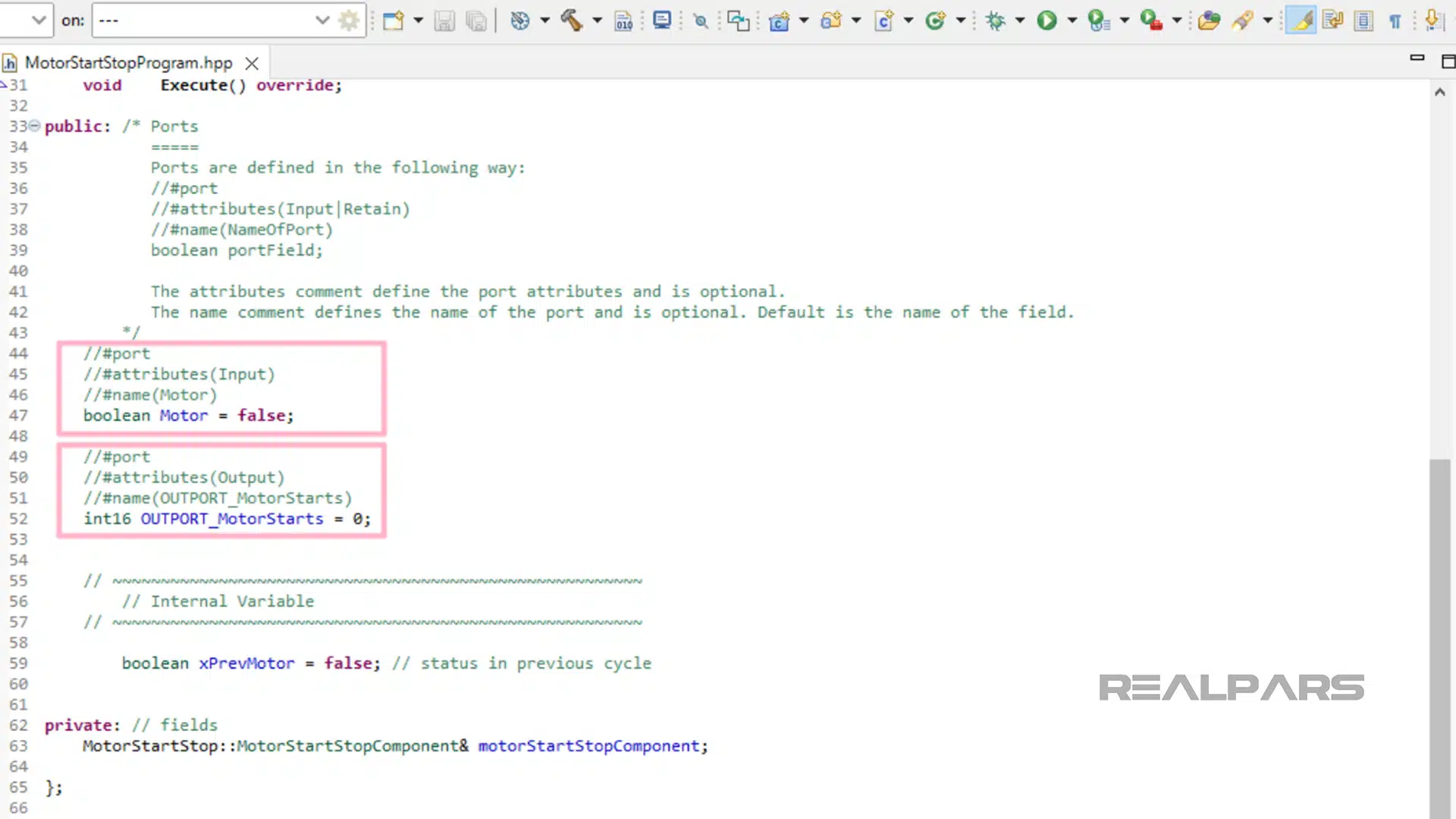
The ports allow the C++ program to communicate with the LD program developed in our PLCnext Engineer programming software.
In a nutshell, an Input Port reads data in, and an Output Port writes data out.

We have an internal BOOLEAN type variable called xPrevMotor.
An internal variable is used within the C++ program only and does not communicate with the external LD program.
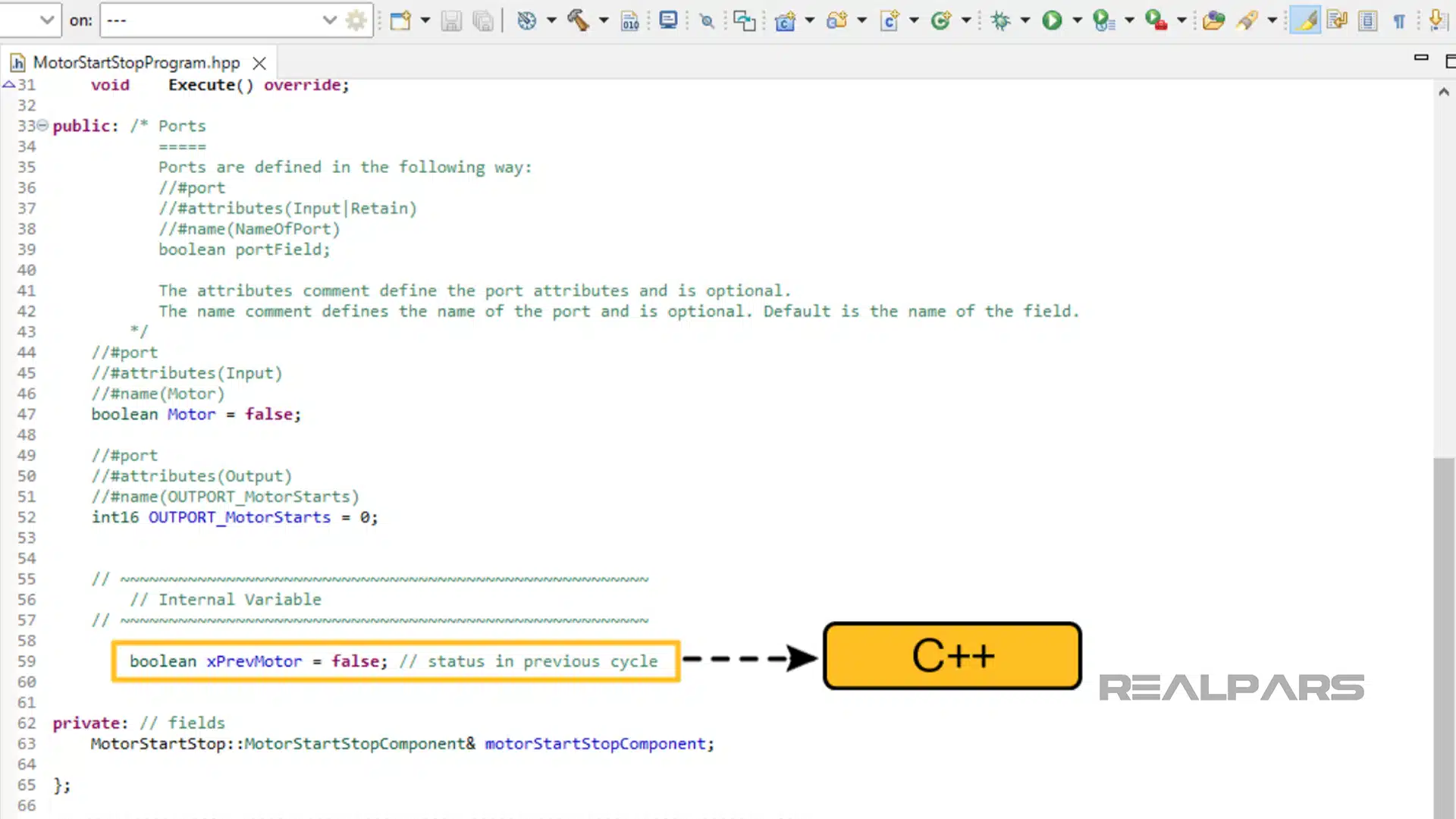
C++ source code file
Ok… and now let’s look at the MotorStartStopProgram.cpp file.
xPrevMotor is an internal variable and is only used within the C++ program.
We know that Motor is assigned to an INPUT Port.
Let’s start with line 10.
Line 10 performs a logical operation comparing the Motor state with its previous state.
– The exclamation mark (!) represents a logical NOT operator.
– The double ampersand (&&) represents a logical AND.
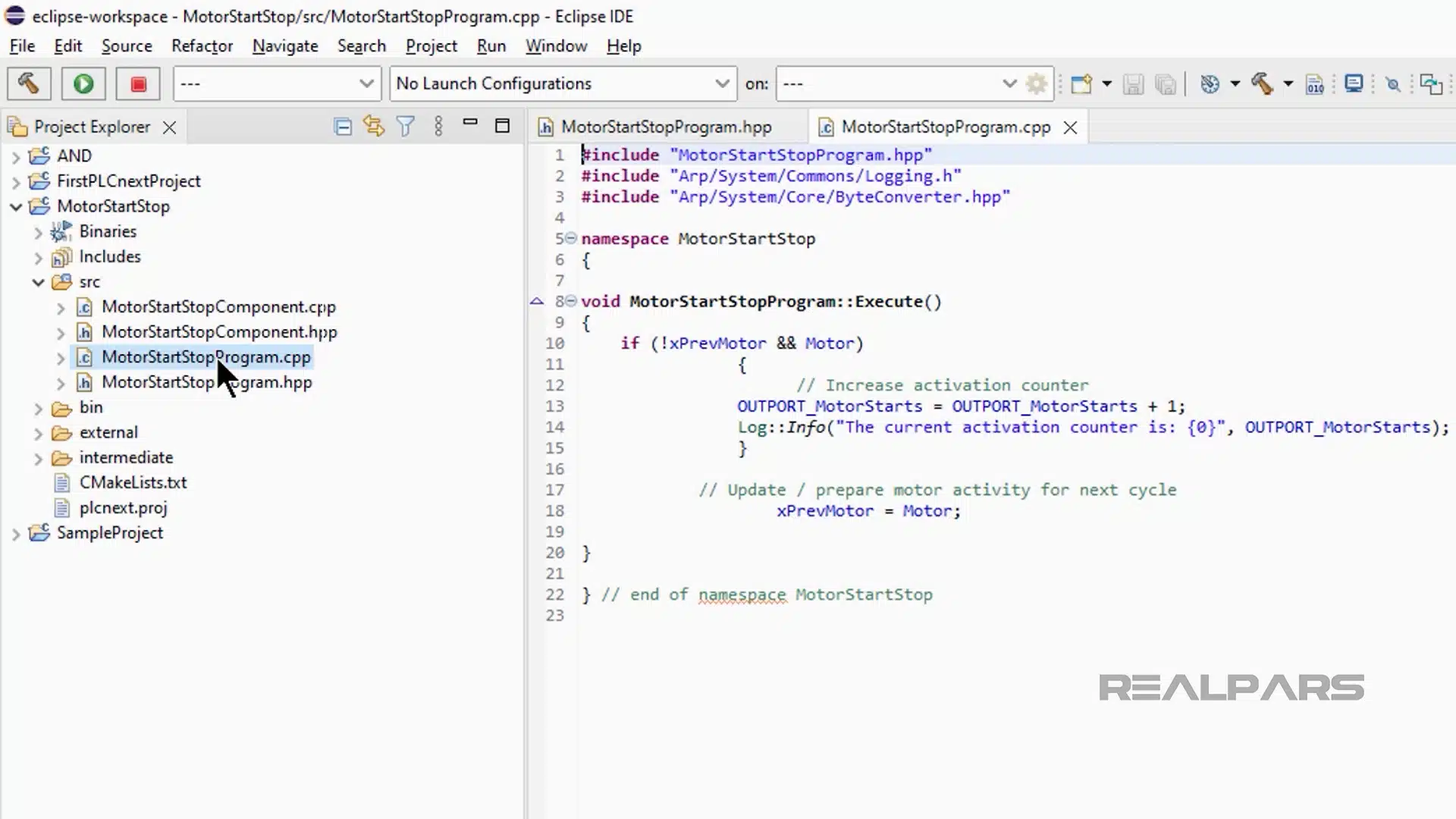
When the Motor changes state from False to True, or OFF to ON, line 13 causes the OUTPORT_MotorStarts int16 value to increment by a count of 1.
The Line 14 statement provides the call to produce the logged data. We want to know when the Motor is activated and how many times it is activated.
The log call will produce a log entry in an output log file each time the activation counter increments.
The log file is called Output.log and is created by PLCnext Technology.
We are using WinSCP to extract and view the contents of the log file. WinSCP is a secure file transfer protocol (SFTP) client software.

Line 18 will set our Boolean xPrevMotor state to be the same as the Motor state in preparation for the next possible change in Motor operation.
Integrating the C++ program with the LD program
Alright… the C++ project is then added to the LD program in PLCnext Engineer.
We know what the Ladder diagram program looks like. Let’s have a peek at the C++ program.
We then run the LD and the C++ programs after we download them to the PLC.
As we operate the START and STOP switches on the Starterkit, the MOTOR will START, then STOP. Each time the Motor cycles, the Out Port value increments.
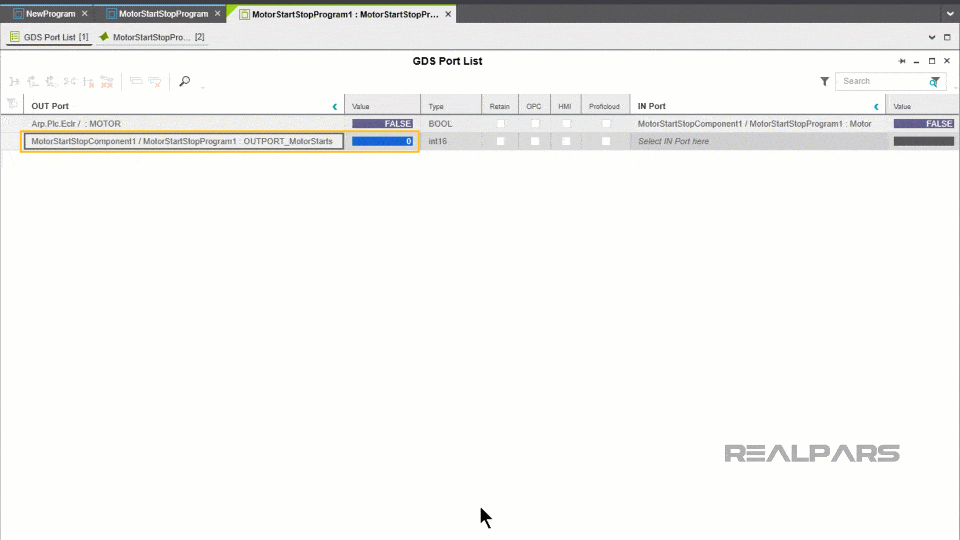
The output log file is opened using WinSCP and we can view the MOTOR activations complete with a Time Stamp.
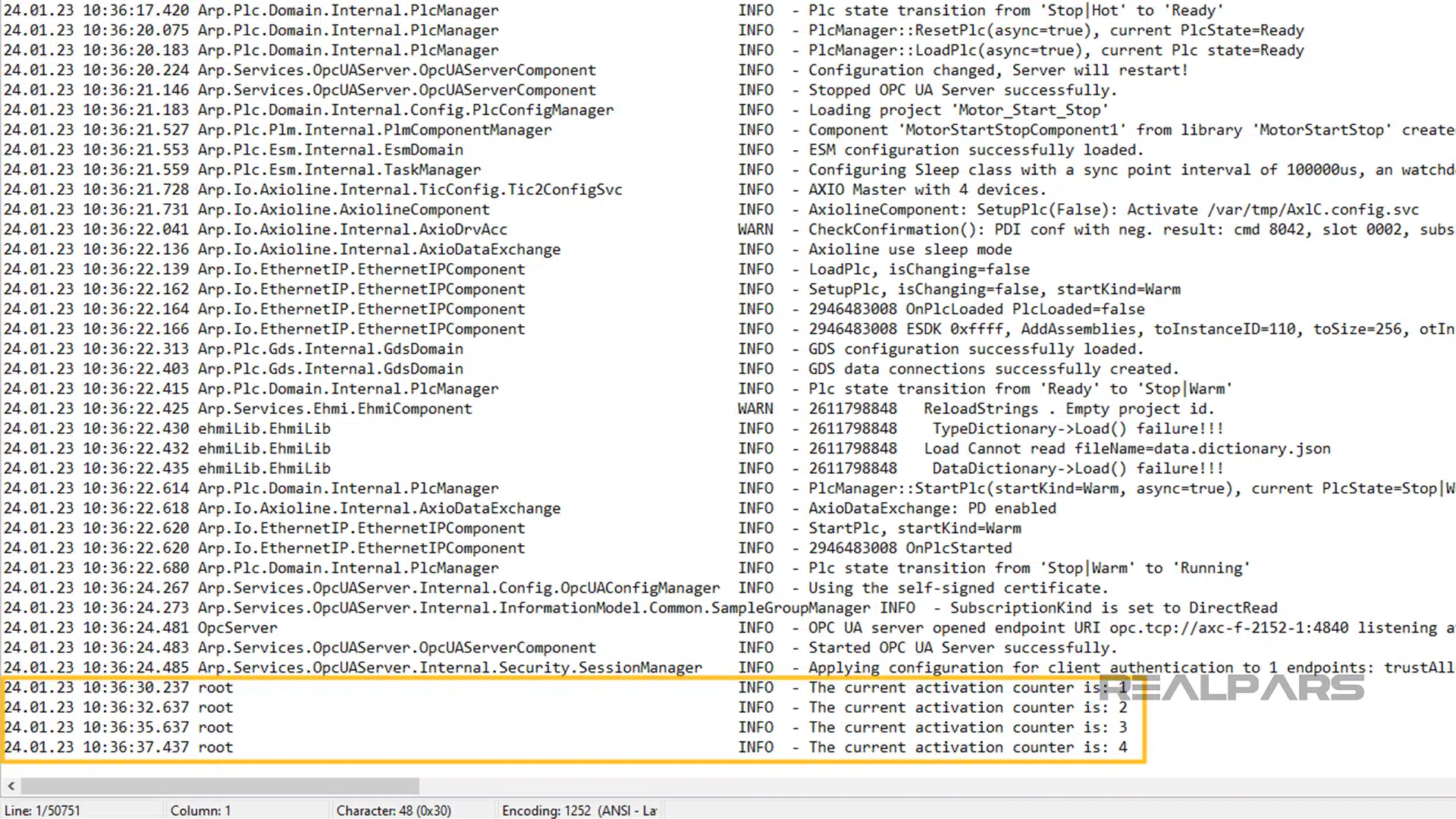
Conclusion
That’s a quick look at PLC Programming with C++.
As we said earlier, C++ programs are used to solve complex functions not easily accomplished with traditional IEC 61131-3 languages.
There are limitless possibilities for using higher-level languages as we unleash more of the PLC’s muscle.
Ready to level up in PLC programming? Unlock the power of C++ for your industrial applications with our Using C++ Projects with PLCnext Technology course – exclusive for RealPars Pro Members.
Get a certificate from Phoenix Contact upon completion, and you’re on your way to becoming a master programmer!